Cubbie Micro
Small. Stupid. Simple. State Storage.
State shouldn't be a chore, keep your state in Cubbie's store
The Lowdown
Cubbie allows the creation of state stores. Cubbie micro differs from cubbie in that it will only keep track of the current and previous state. It is built on an event system that allows anybody to listen for any state event. Event namespacing is available to keep things manageable as lots of events are added.
Installation
npm i -S cubbie-micro
or
yarn add cubbie-micro
Usage
Creating a Store
const cubbie = ;const store = cubbie;
Each store you create is independent, each managing its own state history. So if you want multiple smaller stores in your app rather than one large store, Cubbie will allow it.
const heroes = cubbie;const villains = cubbie;
Initial State
Before you can start tracking state, you need to set an initial state:
store;
initialState
triggers the STATE_SET
event. See the Events section.
To get the initial state:
storeinitialState;
Current State
Accessing the current state is as simple as accessing the state
property.
storestate;
Modifying State
This is the key to Cubbie's simplicity. To modify state, just pass a function to modifyState
. The only parameter of that function is the new state that you get to modify before it is set as the new state.
store;
This is an immutable operation and the newly modified state object will become the current state, and what was the current state will become the previous state.
modifyState
triggers the STATE_MODIFIED
event. See The Event System.
modifyState
will return the new current state.
State History
Not available in Cubbie Micro (only in Cubbie Original)
Get Previous State
This returns the state immediately before the current state.
storepreviousState;
Views
Similar in purpose to SQL views, views allow you to store a function that you can call on at any moment. Its just some good ol' logic abstraction.
Example (using lodash's maxBy
)
console; /*{ people: [{name: 'cat', age: 13}, {name: 'sam', age: 25}, {name: 'jas', age: 20}]}*/store;store; // {name: 'sam', age: 25}
The Event System
See The Event System
Usage with React
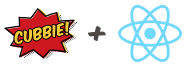